CS106L笔记:Multi-threading
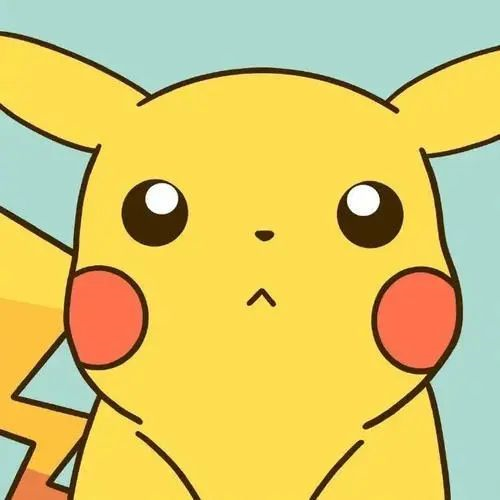
Multi-threading
Thread
- threads are ways to parallelise execution
what’s the problem?
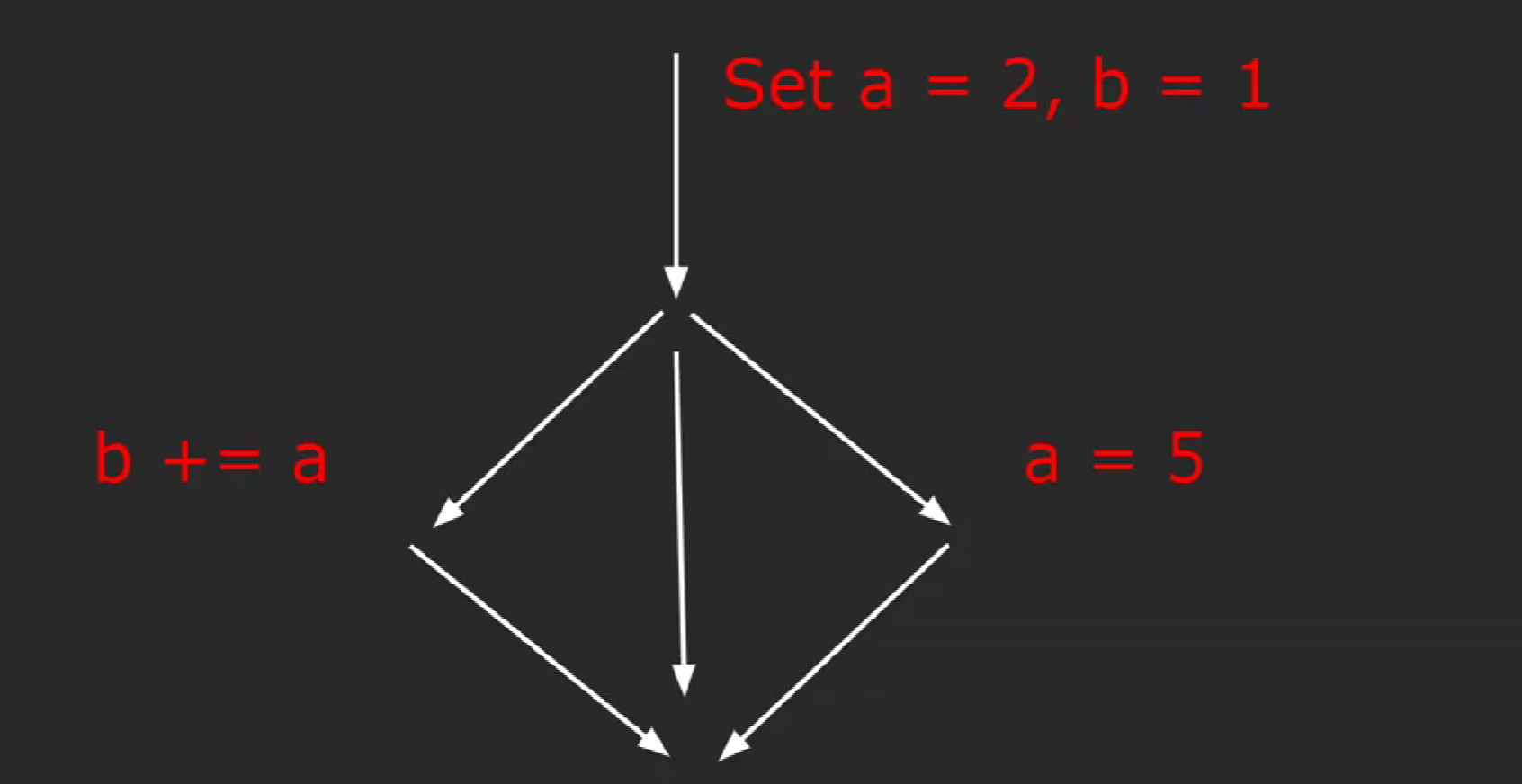
DATA RACE!
use LOCK!
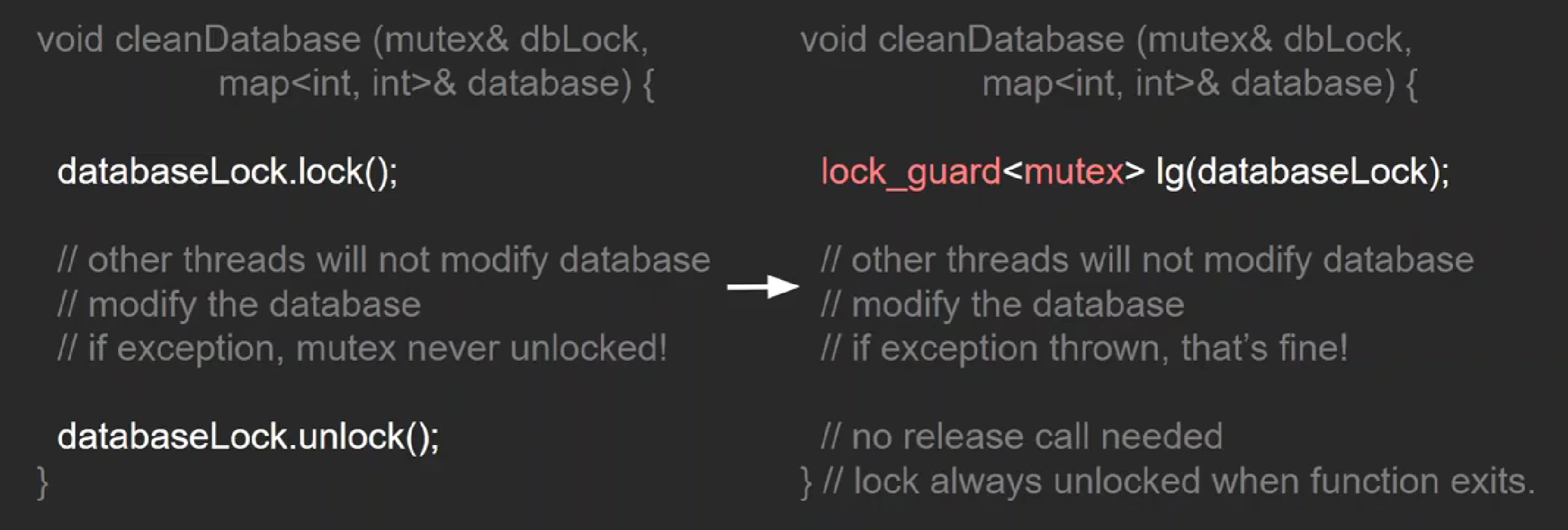
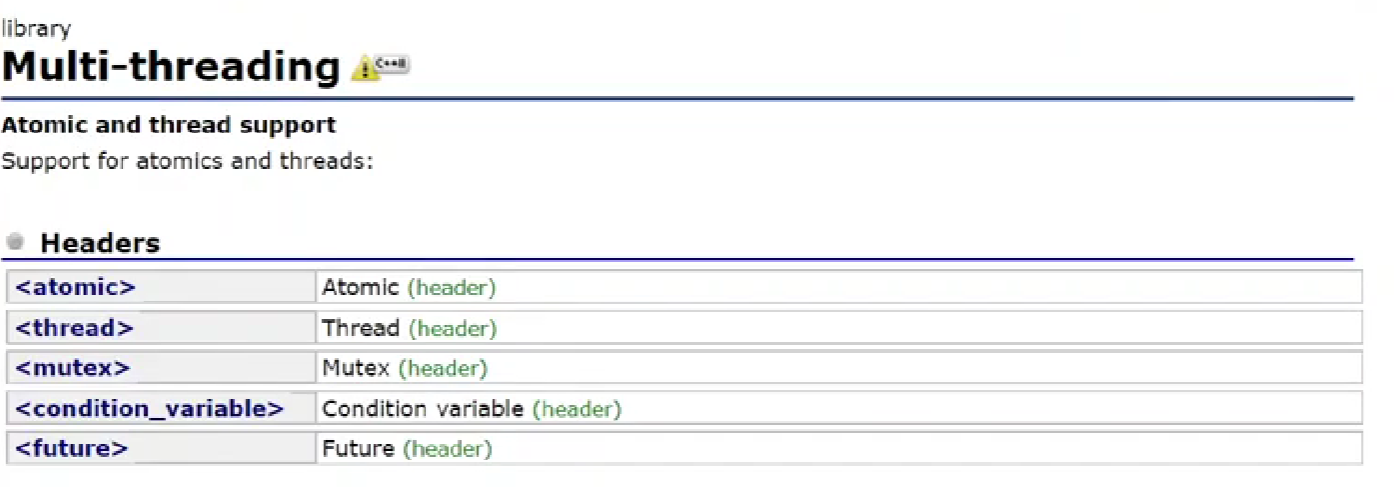
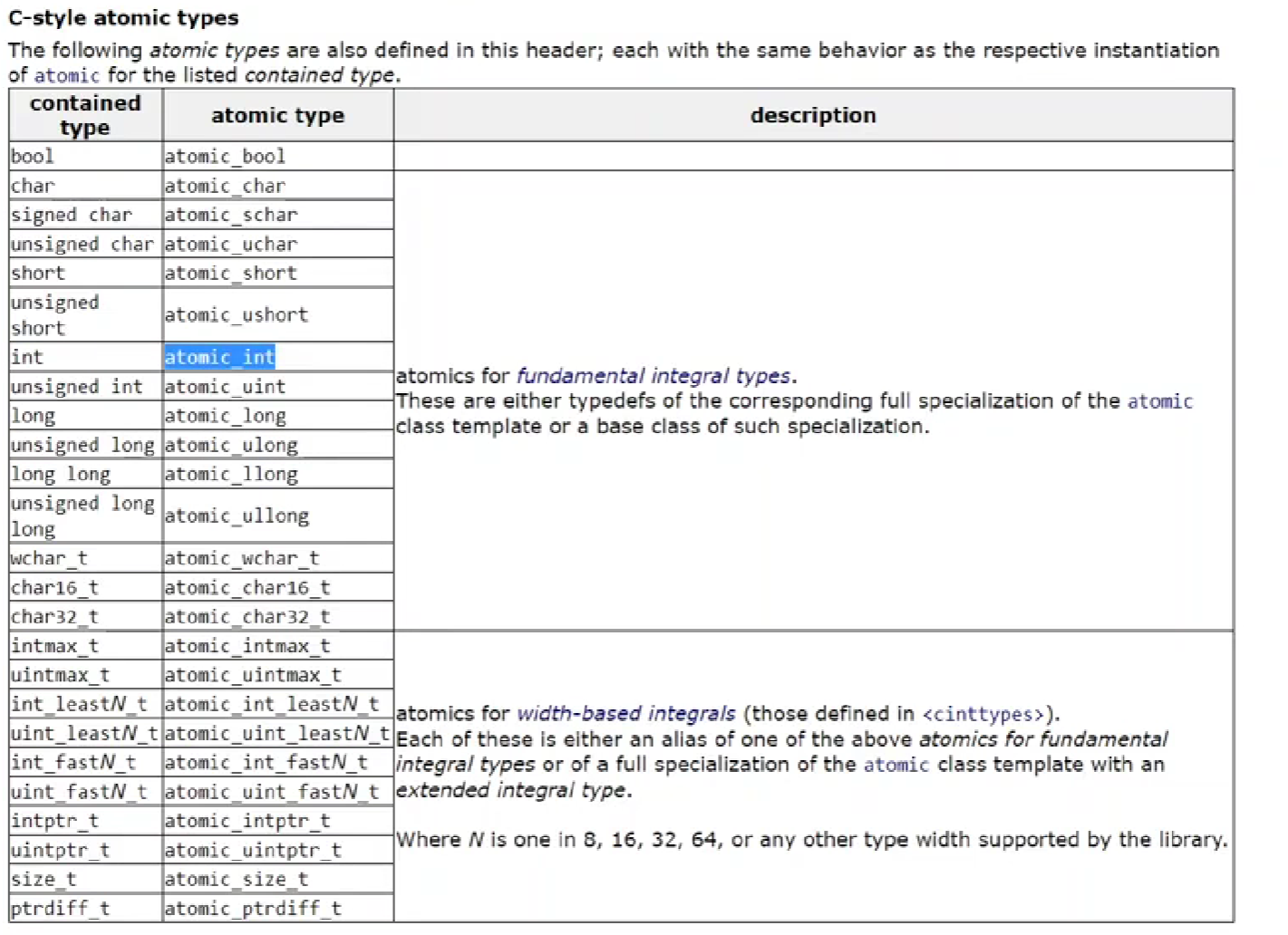
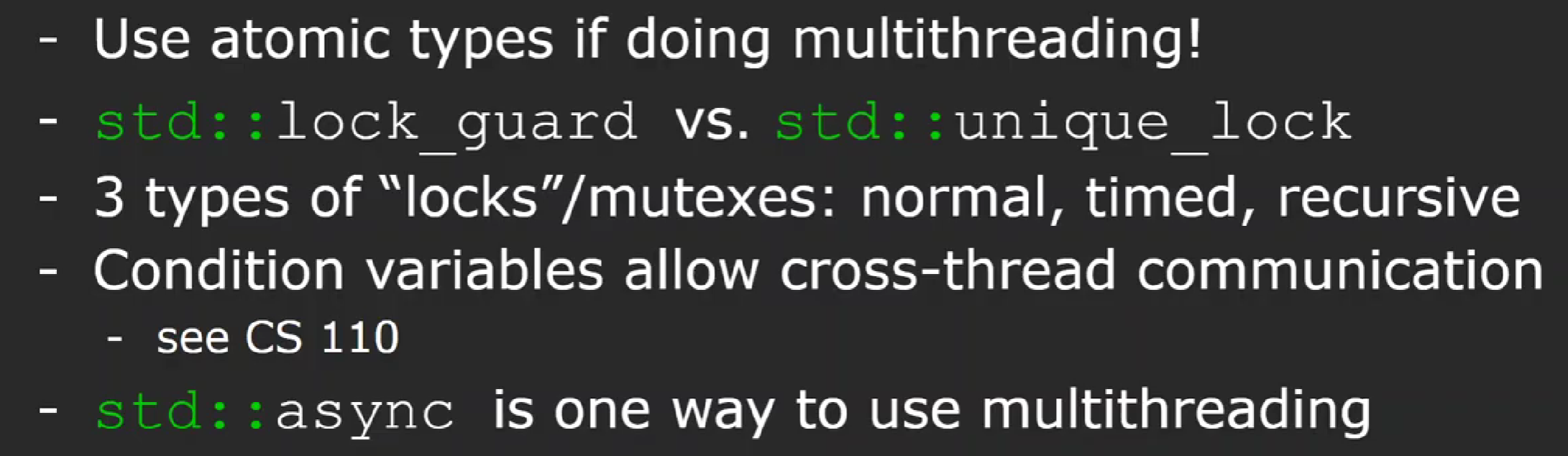
1 | //std::mutex mtx; |
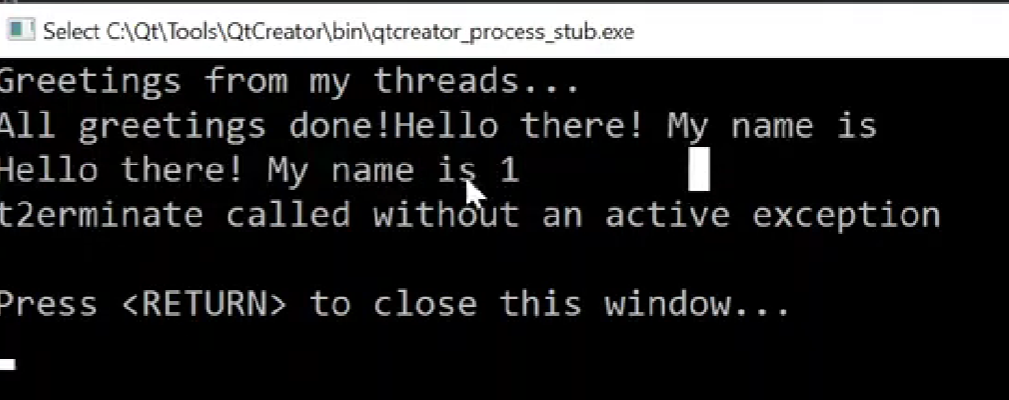
- Data Racing is unpredictable!
use lock_guard to ensure the greeting is atomic:
1 | std::mutex mtx; |
use join to let the main wait the thread to finish
1 | thread1.join(); |
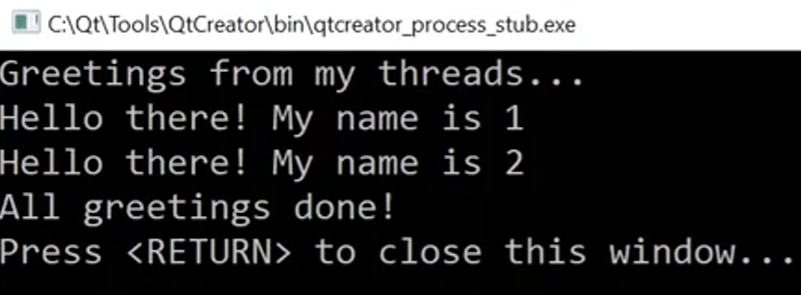
- the results seems fine!
1 | //...work with vector if threads |
- Post title:CS106L笔记:Multi-threading
- Post author:sixwalter
- Create time:2023-08-05 11:14:26
- Post link:https://coelien.github.io/2023/08/05/course-learning/CS-106L/Multi-Threading/
- Copyright Notice:All articles in this blog are licensed under BY-NC-SA unless stating additionally.
Comments