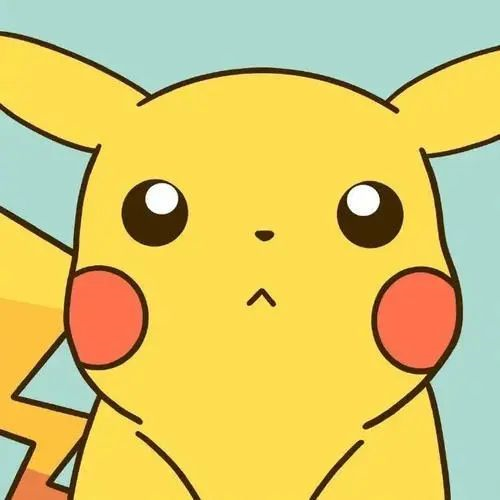
Template Metaprogramming
Motivating example
1 |
|
how do you implement your own distance function?
1 | template<typename It> |
- the above code is O(1), which is efficient.
- but the above implementation only works for random access iterator
another optimization!
1 | template<typename It> |
- but the second way is O(N), for any kind of iterators, which is unexpected
How does STL achieve this?
- You have to extract type information from iterator of what it is.
- need to determine if it is random access iterator
- run the piece of code based on the type of iterator:
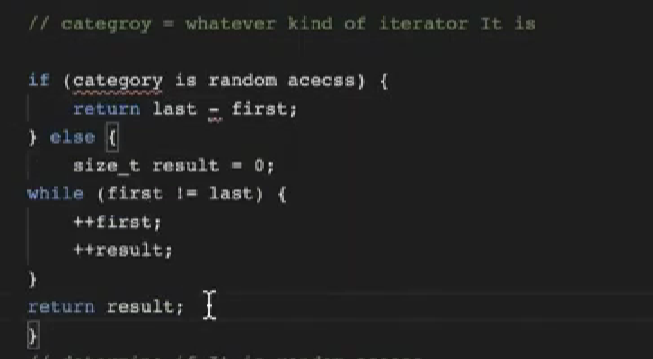
Computations on Types
Comparision of values vs. type computations : A Preview
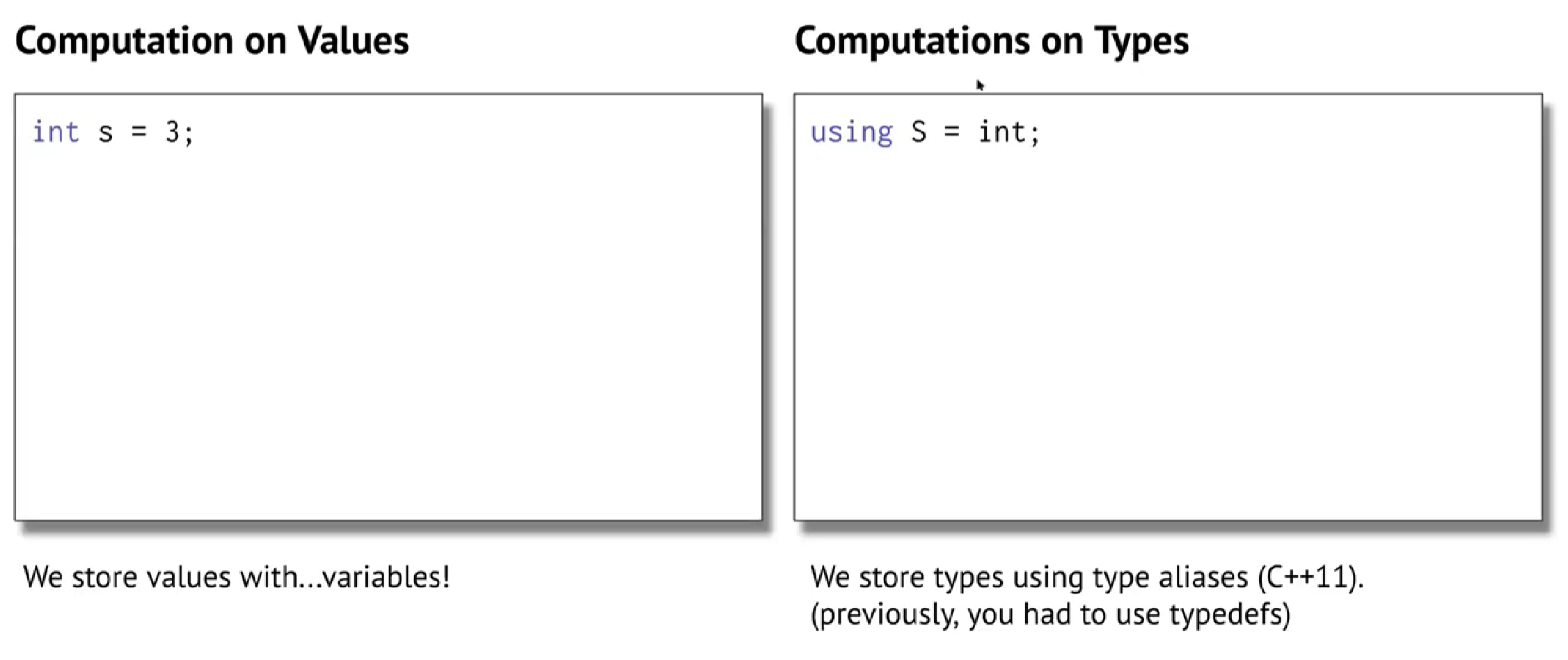
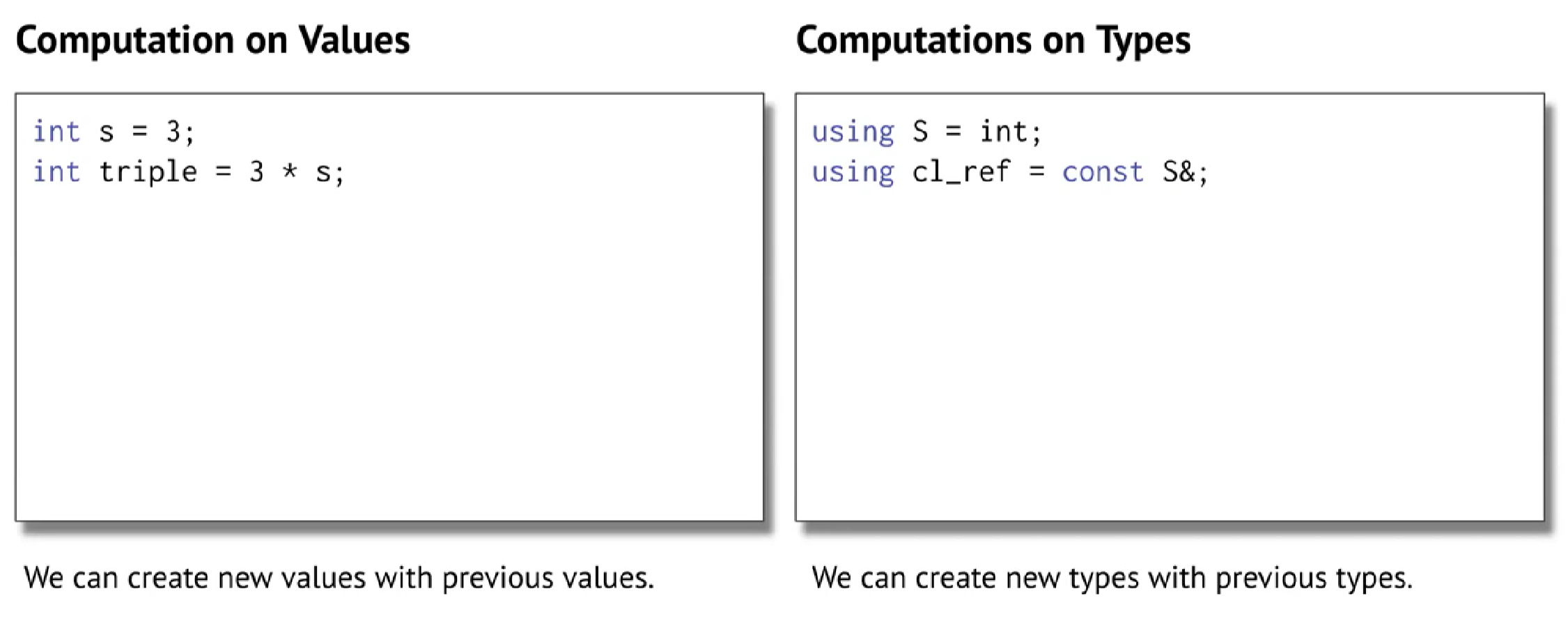
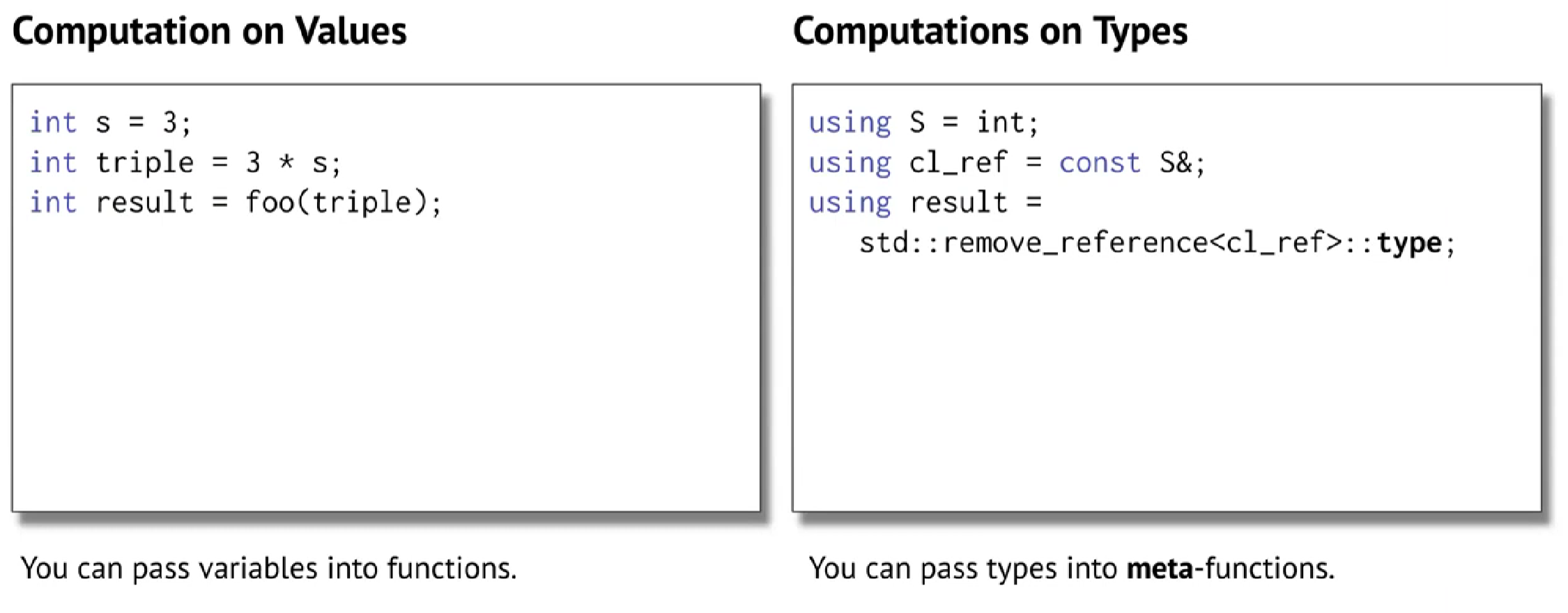
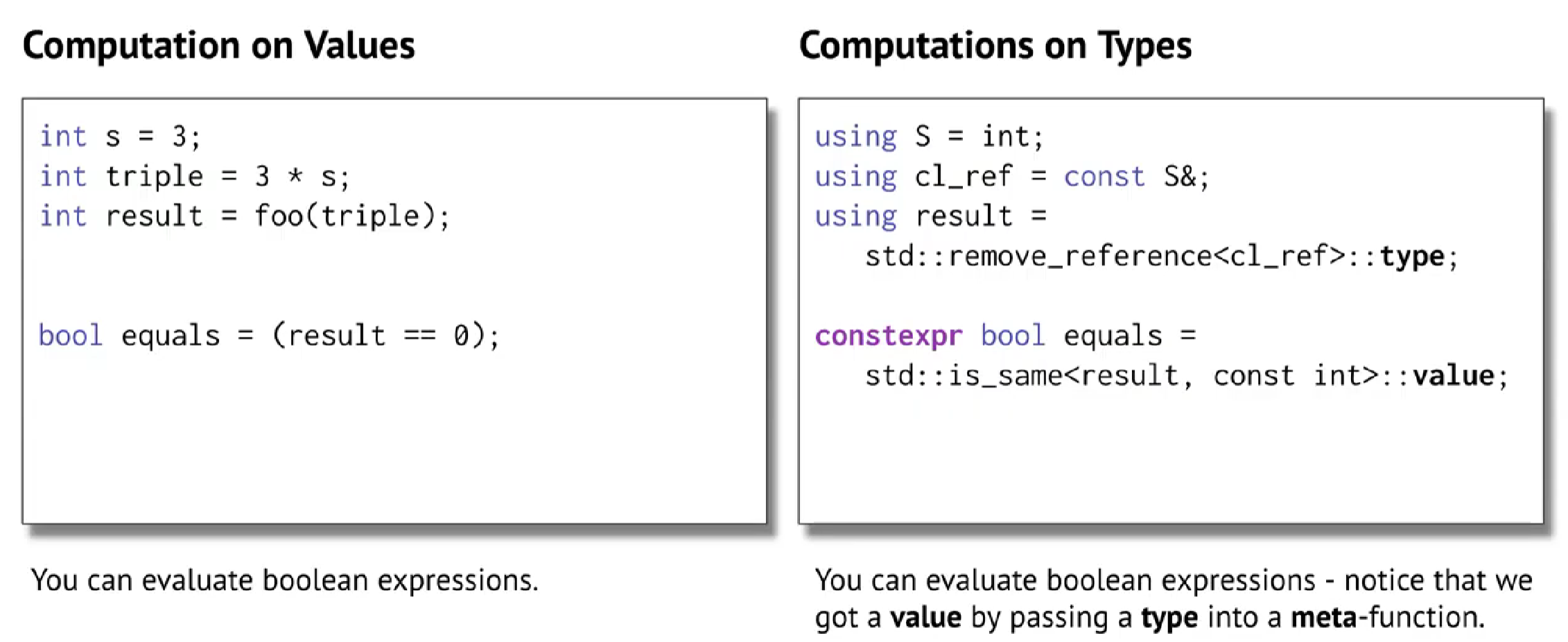
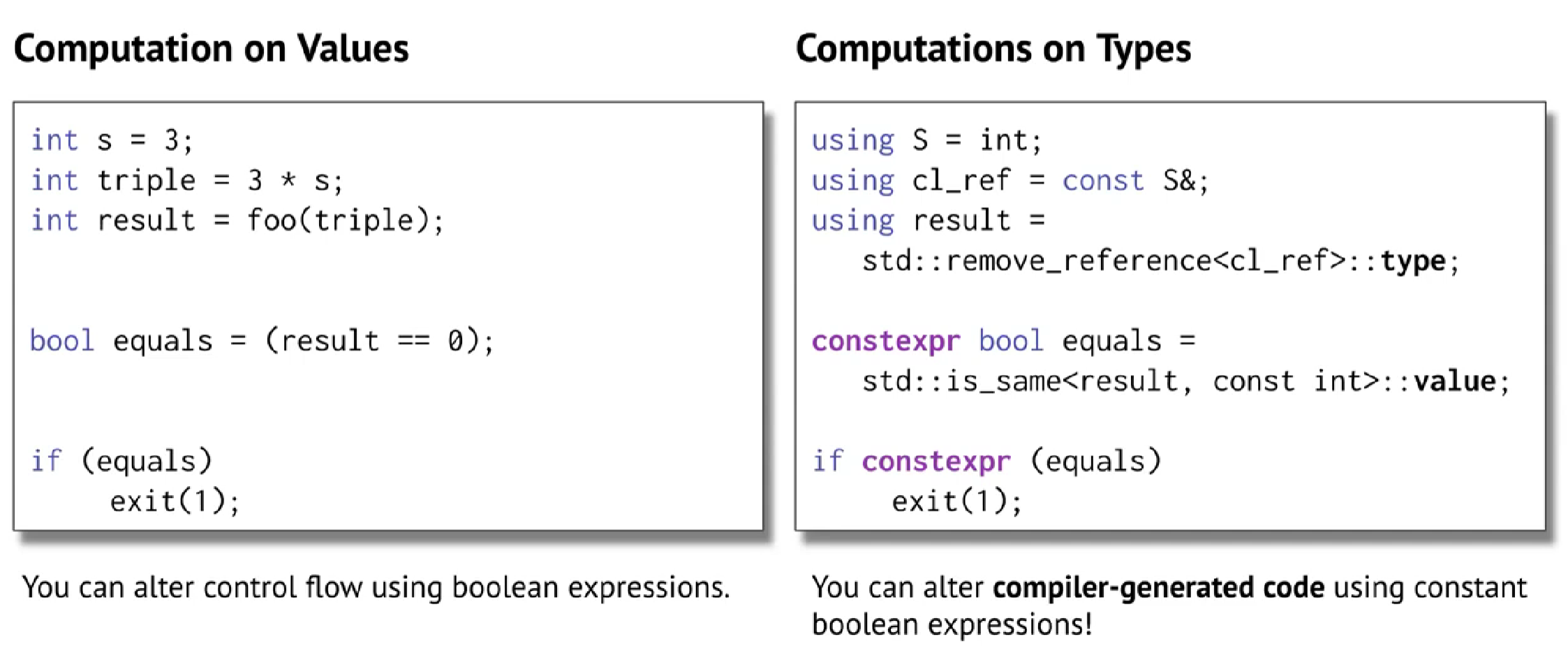
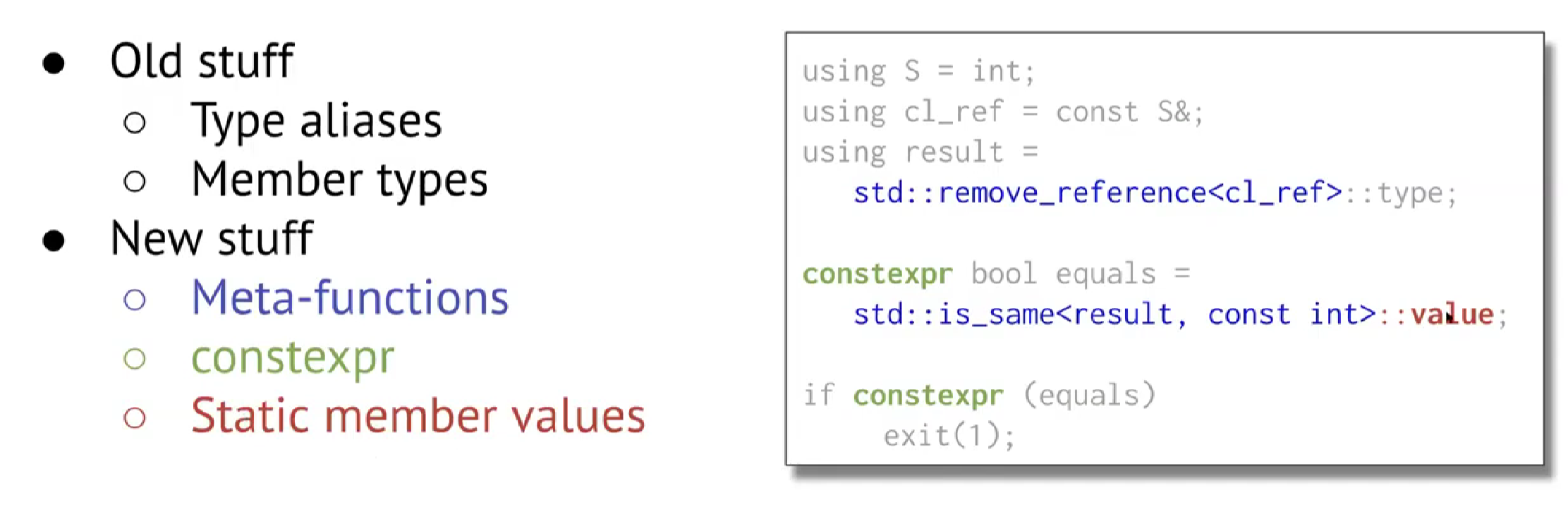
Meta-functions
template types
- use them for template classes
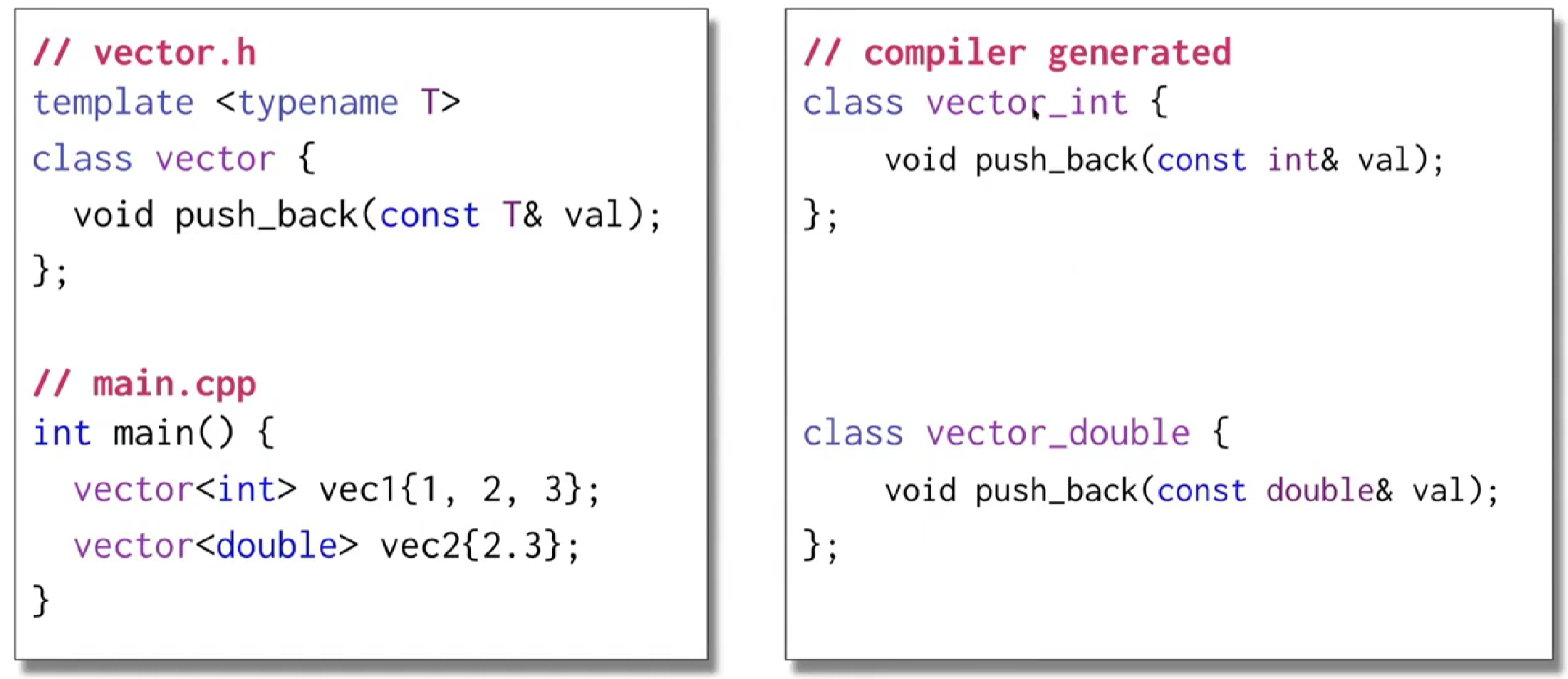
template values
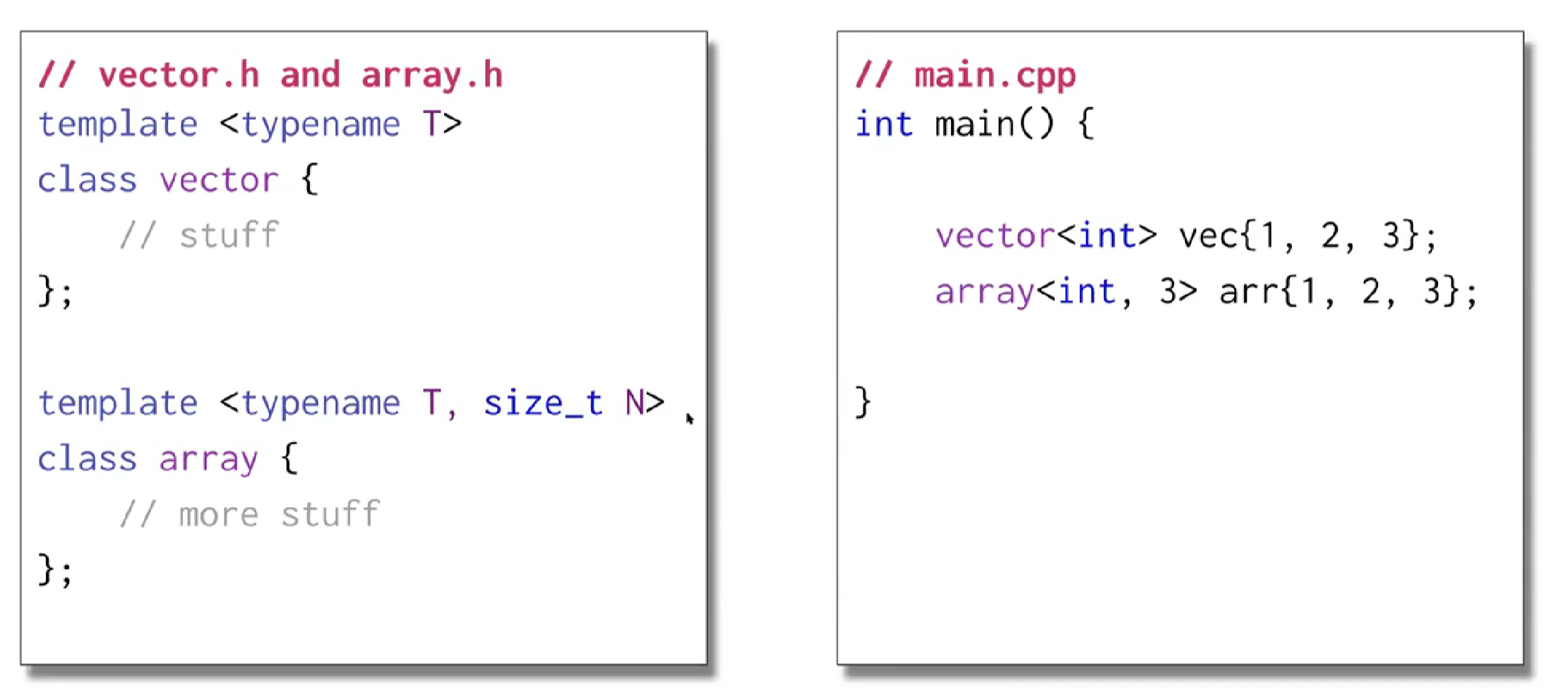
meta-function
abstractly:
- It is a function that operates on some types/values(“parameters”)
- and outputs some types/values(“return values”)
concretely:
- It is a struct that has public member types/fields(output) which depend on what the template types/values(input) are instantiated with.
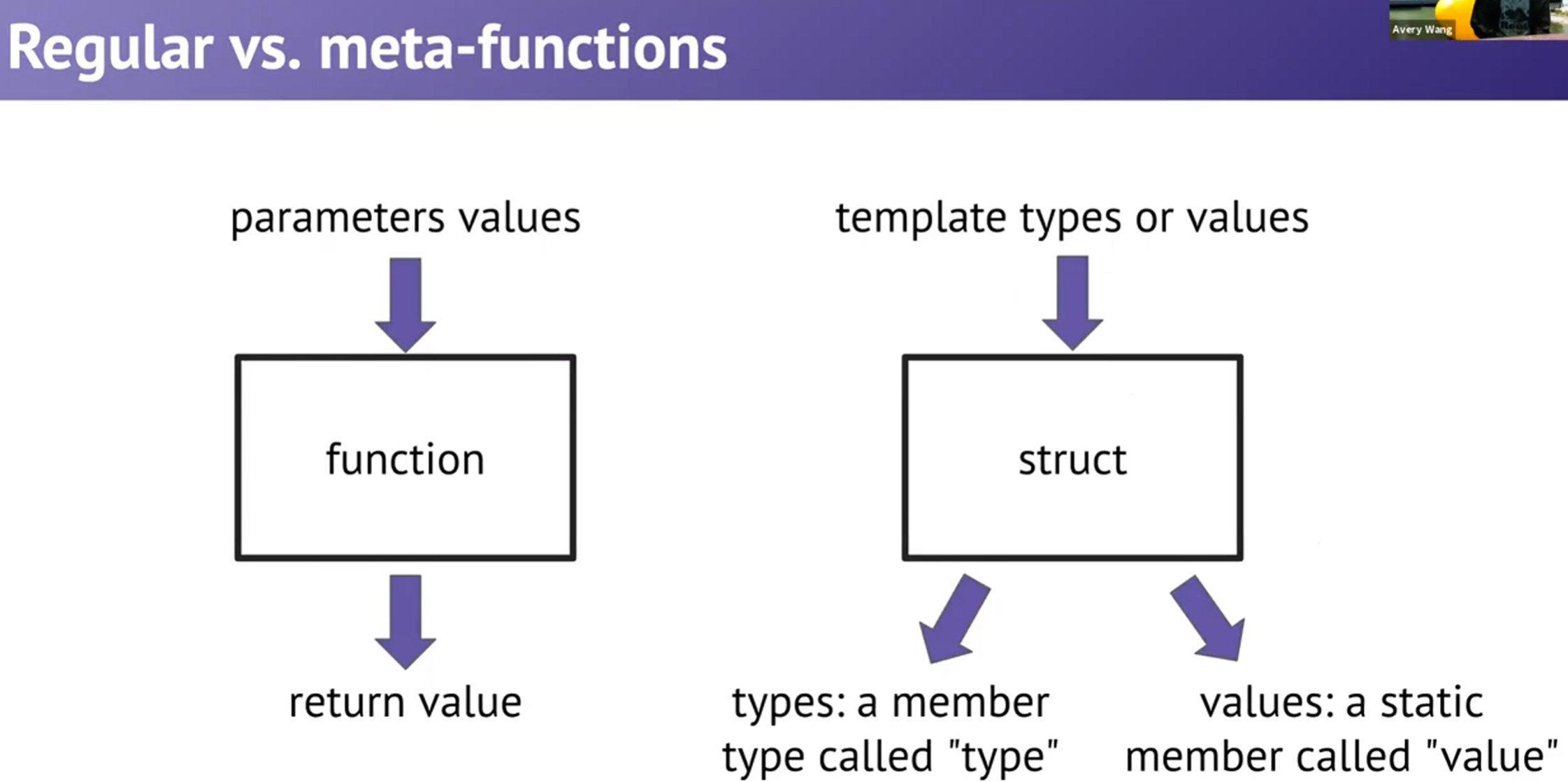
- a simple example:
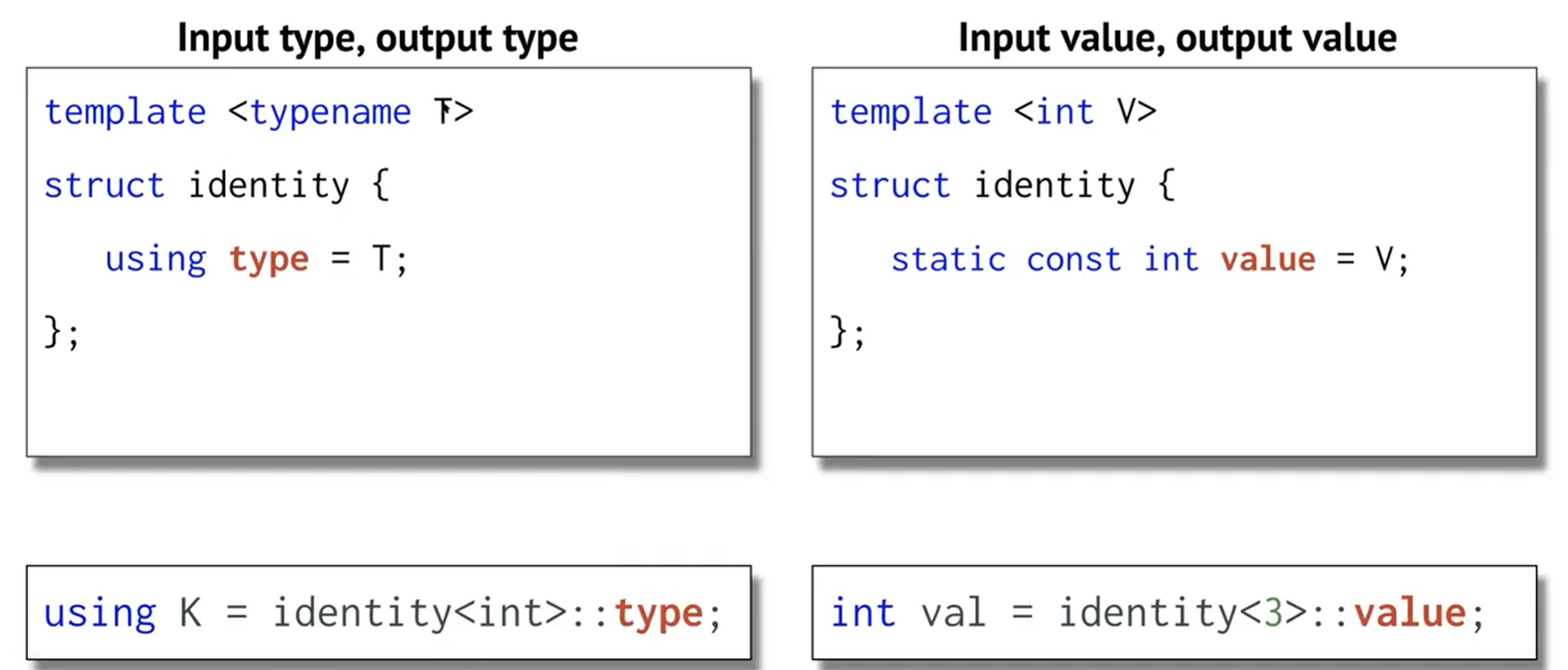
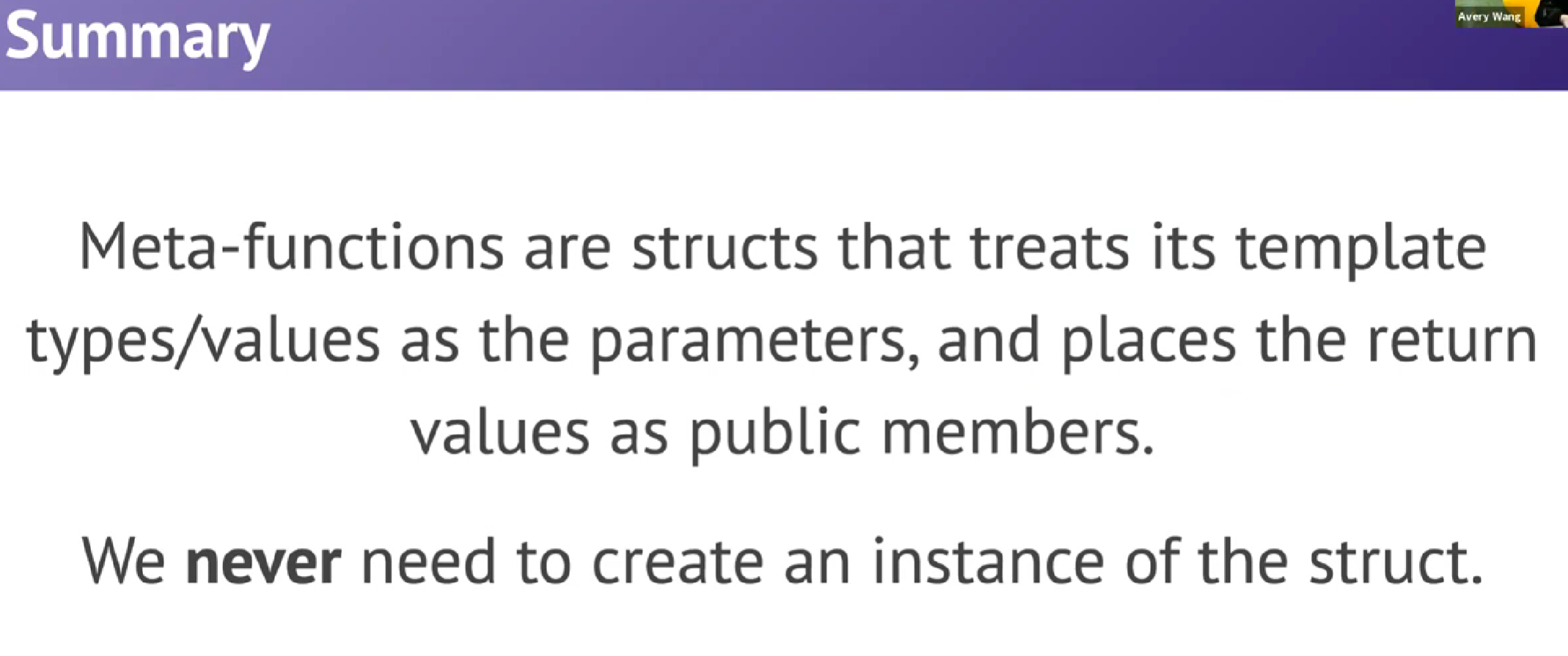
1 | //input type, output value |
Template Deduction
Template Rules
- Template Specialization: you can have a “generic template”, as well as “specialized” templates for particular types
Fully specialize:
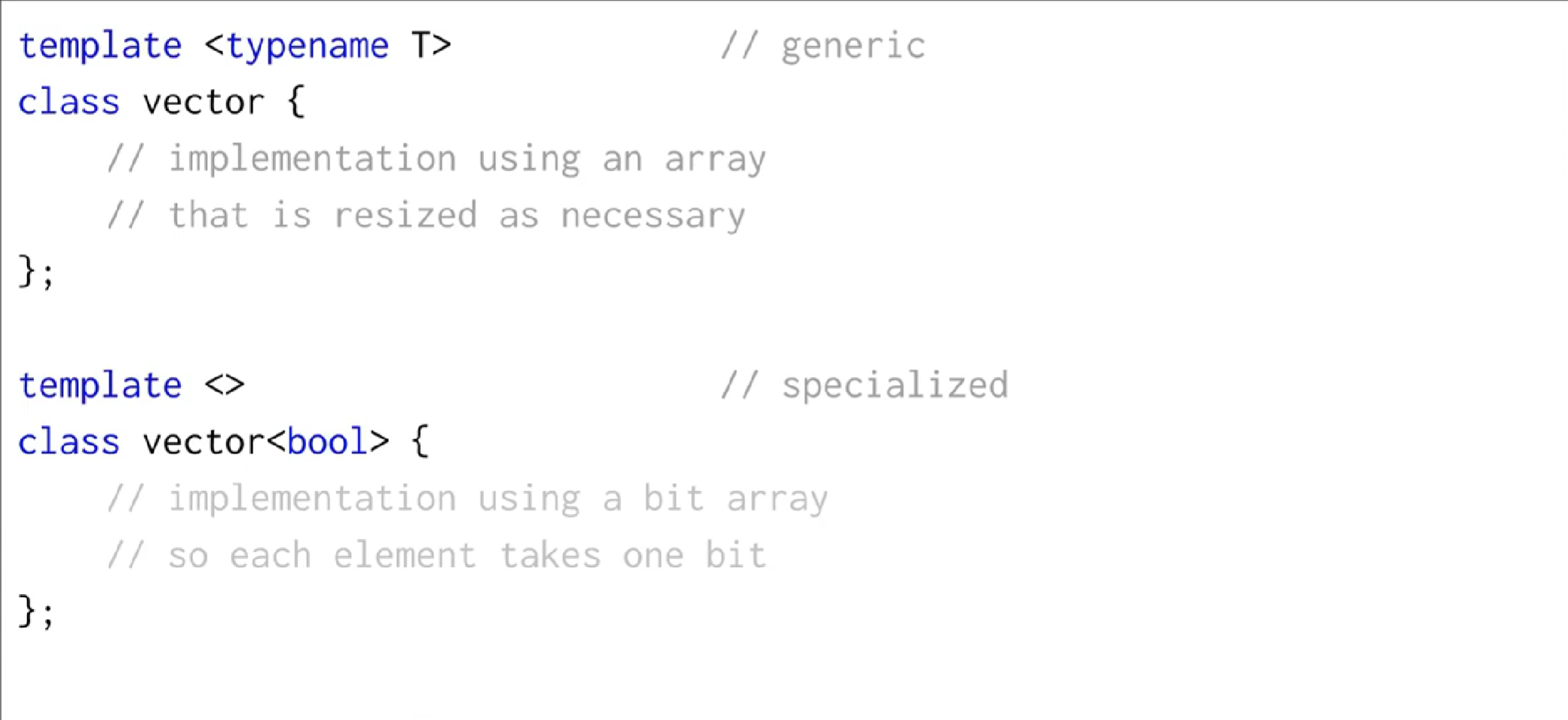
why specialization?: using a bit array to store booleans is much more efficient.
partially specialize:
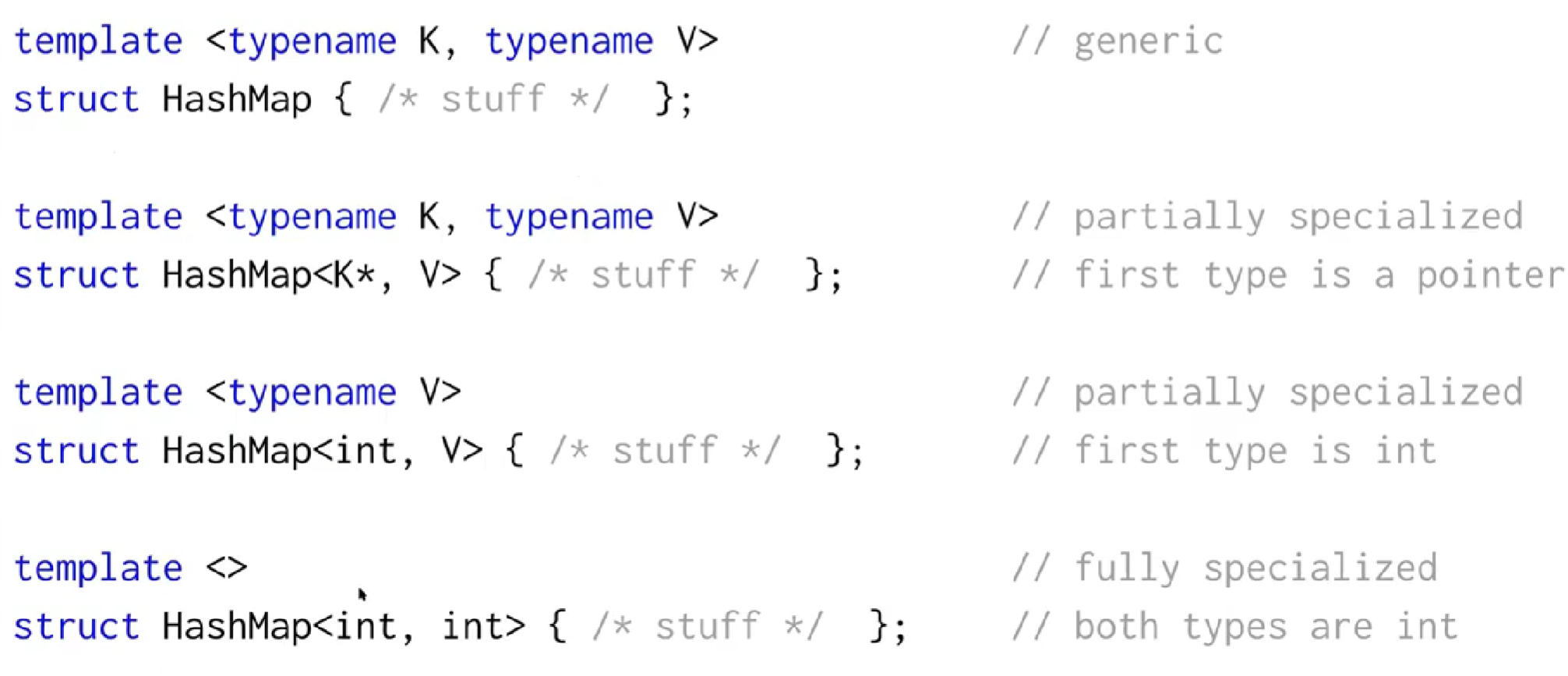
Some examples using Template Deduction
let’s build up a collection of predicate meta-functions
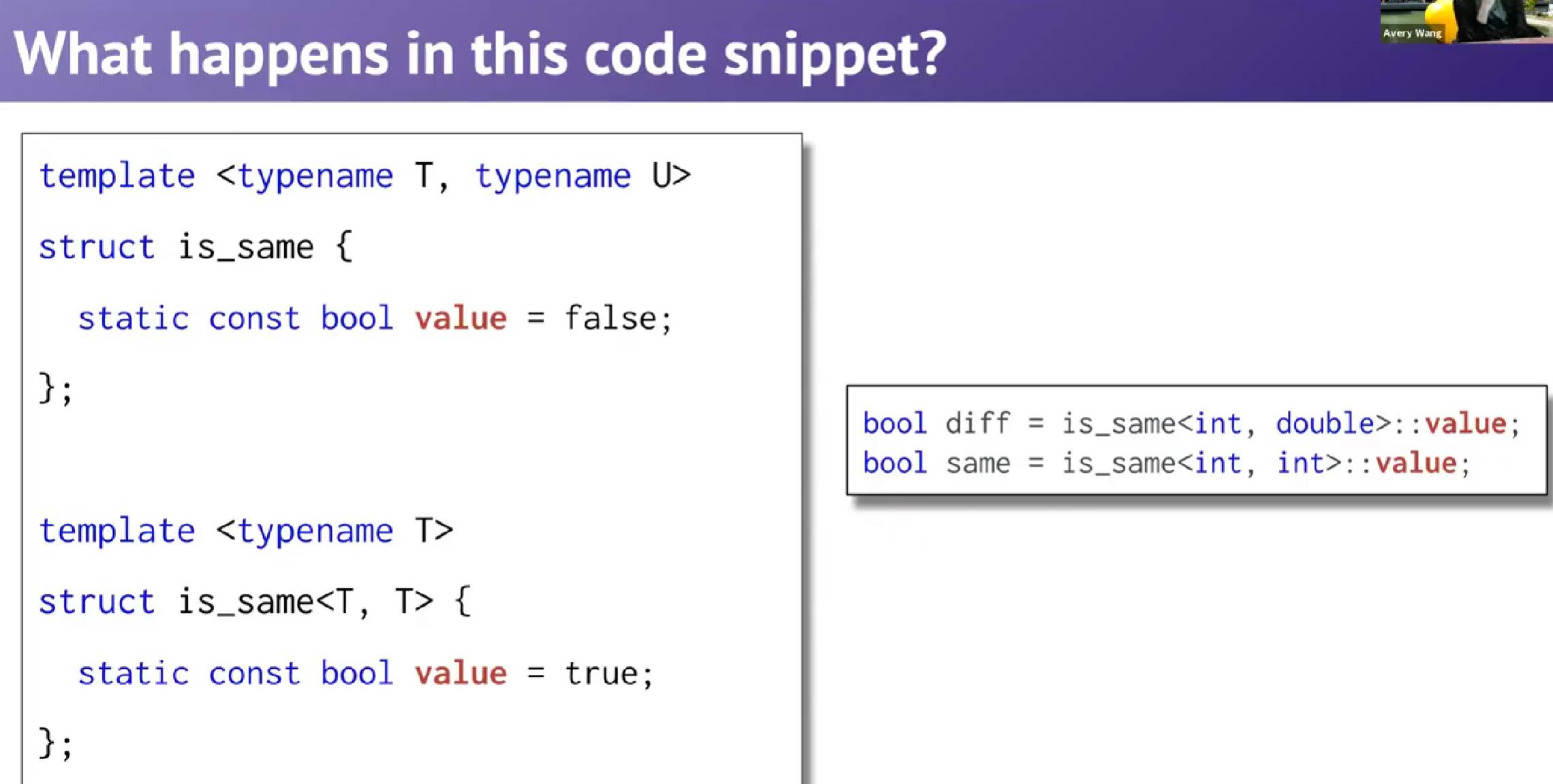
why does the second one return true?
- the compiler tries to first match the specialized template
- the second one got matched, so return true
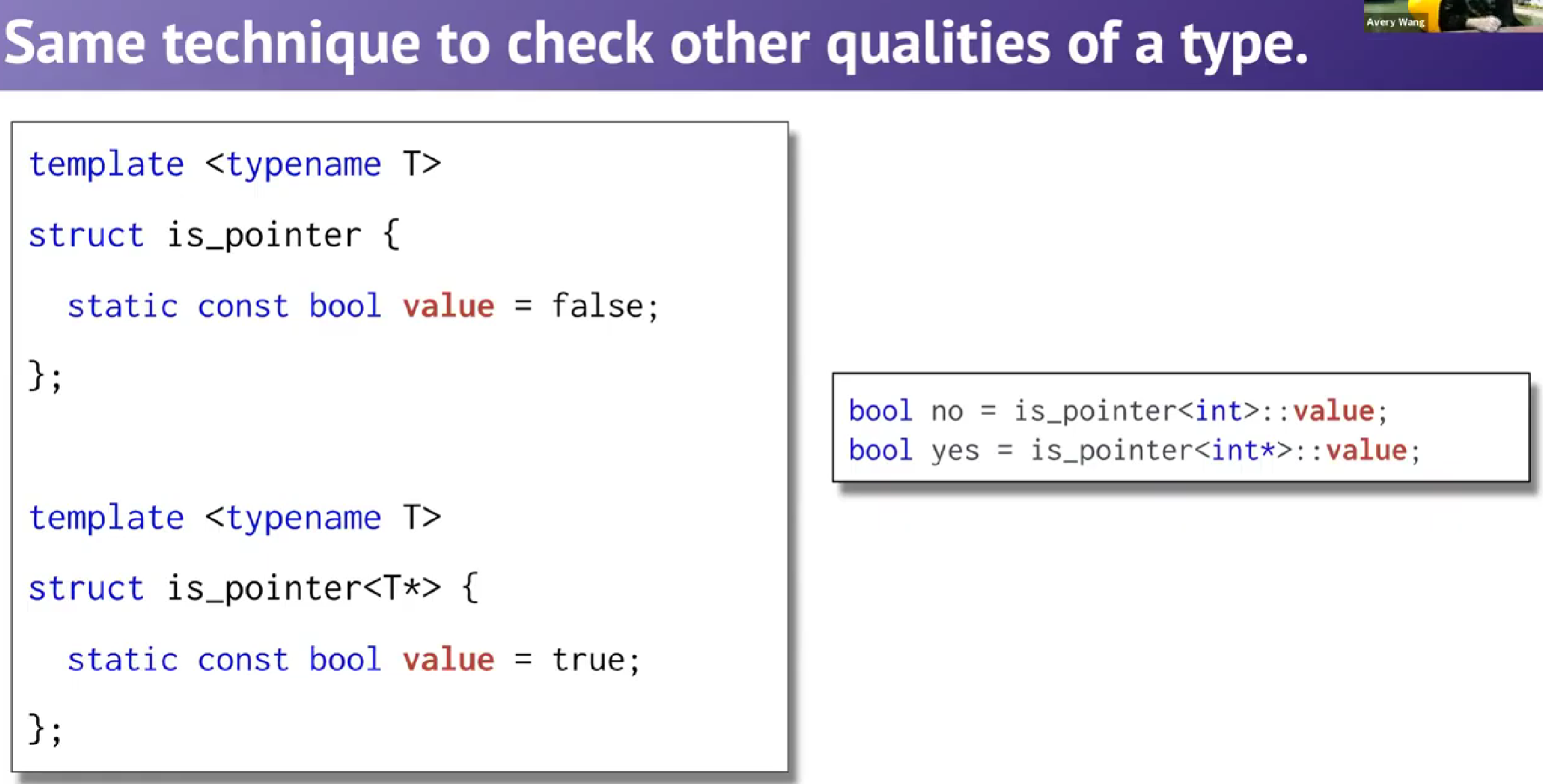
- kind of interesting, you can figure out the type info, because of template deduction rules
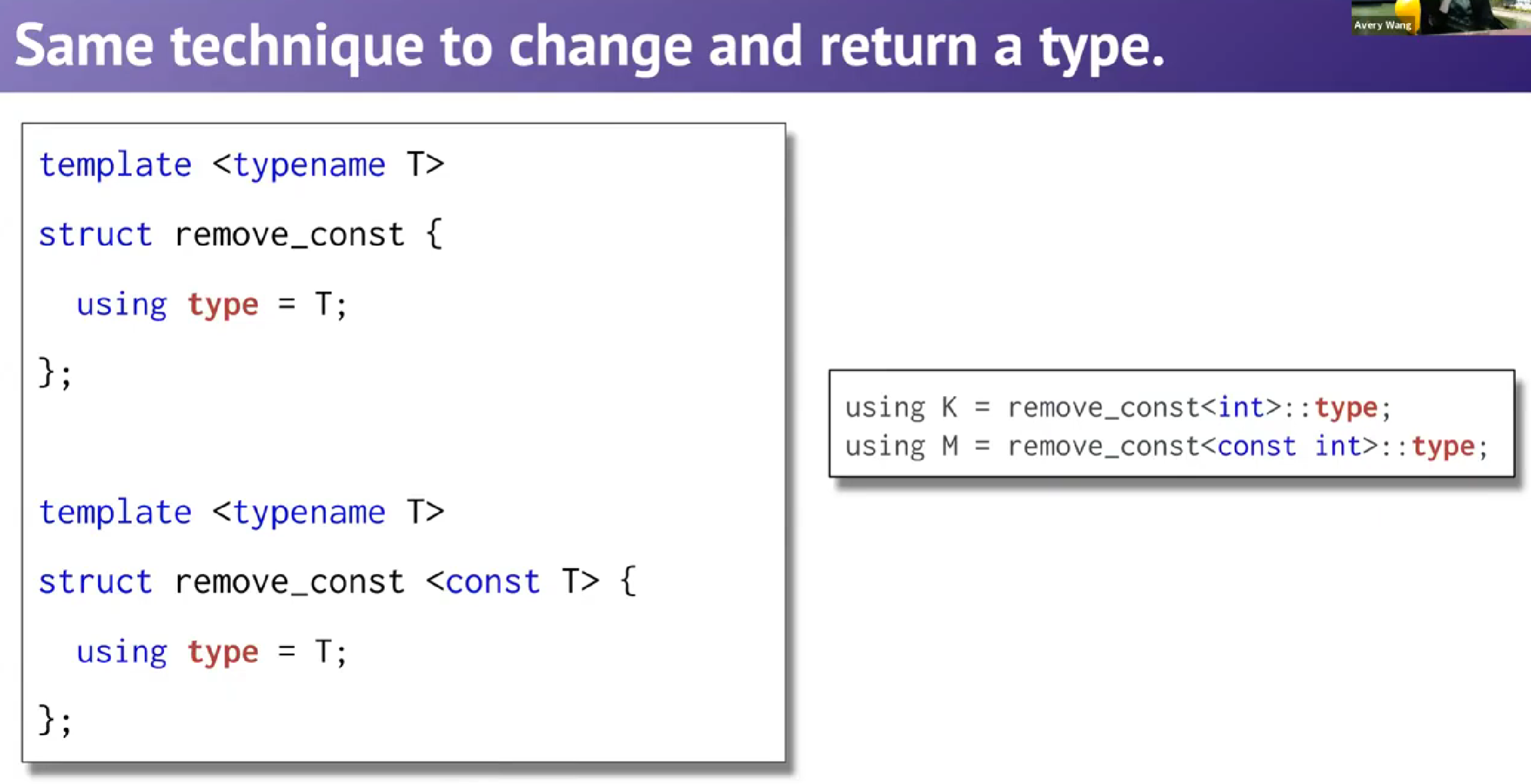
- of course, same technique can be used to return a type
Actually it is a “hack”, because we are using the compiler’s template matching rules to implement an if/else statement for types(we can’t directly compare them)
Let finish our myDistance implement:
1 | using category = typename std::iterator_traits<It>::iterator_category; |
need to remove the offending code when the if statement knows that part won’t be run;
pre-C++17: std::enable_if
C++17: if constexpr
1 | using category = typename std::iterator_traits<It>::iterator_category; |
what is constexpr?
you can calculate this expression at compile time.(stronger form of const)
what is if constexpr?
calculate boolean at compile-time , Replace the entire if/else with the code that will be actually be run
- Post title:CS106L笔记:Template Metaprogramming
- Post author:sixwalter
- Create time:2023-08-05 11:14:26
- Post link:https://coelien.github.io/2023/08/05/course-learning/CS-106L/Template_Metaprogramming/
- Copyright Notice:All articles in this blog are licensed under BY-NC-SA unless stating additionally.